In this tutorial, we will learn how to implement role-based authorization using Spring Boot 3, Spring Security, and MySQL database. We will use two roles - ADMIN, and USER.
We will build the REST APIs and secure them using Spring Security's role-based authorization.
Spring Security is a framework that provides authentication, authorization, and protection against common attacks. With first-class support for securing both web and reactive applications, it is the de-facto standard for securing Spring-based applications.
1. Add Maven Dependencies
Add below Maven dependencies to your Spring Boot project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
2. Configure MySQL Database
Let's first create a database in MySQL server using the below command:
create database login_system
Since we’re using MySQL as our database, we need to configure the database URL, username, and password so that Spring can establish a connection with the database on startup. Open the src/main/resources/application.properties file and add the following properties to it:
spring.datasource.url=jdbc:mysql://localhost:3306/login_system
spring.datasource.username=root
spring.datasource.password=Mysql@123
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
spring.jpa.hibernate.ddl-auto=update
3. Create JPA Entities - User and Role (Many-to-Many Mapping)
In this step, we will create User and Role JPA entities and establish MANY-to-MANY relationships between them. Let's use JPA annotations to establish MANY-to-MANY relationships between User and Role entities.
User
import jakarta.persistence.*;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import java.util.Set;
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false, unique = true)
private String email;
@Column(nullable = false)
private String password;
@ManyToMany(fetch = FetchType.EAGER, cascade = CascadeType.ALL)
@JoinTable(name = "users_roles",
joinColumns = @JoinColumn(name = "user_id", referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "role_id", referencedColumnName = "id")
)
private Set<Role> roles;
}
Role
import jakarta.persistence.*;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@Entity
@Table(name = "roles")
public class Role {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
}
4. Create Spring Data JPA Repositories
Next, let's create a repository package. Within a repository package, we create UserRepository and RoleRepository interfaces.
public interface UserRepository extends JpaRepository<User, Long> {
}
public interface RoleRepository extends JpaRepository<Role, Long> {
Role findByName(String name);
}
5. Spring Security Implementation
CustomUserDetailsService
Let's create a CustomUserDetailsService class that implements the UserDetailsService interface ( Spring security in-build interface) and provides an implementation for the loadUserByUername() method:
import lombok.AllArgsConstructor;
import net.javaguides.todo.entity.User;
import net.javaguides.todo.repository.UserRepository;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
import java.util.Set;
import java.util.stream.Collectors;
@Service
@AllArgsConstructor
public class CustomUserDetailsService implements UserDetailsService {
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String usernameOrEmail) throws UsernameNotFoundException {
User user = userRepository.findByUsernameOrEmail(usernameOrEmail, usernameOrEmail)
.orElseThrow(() -> new UsernameNotFoundException("User not exists by Username or Email"));
Set<GrantedAuthority> authorities = user.getRoles().stream()
.map((role) -> new SimpleGrantedAuthority(role.getName()))
.collect(Collectors.toSet());
return new org.springframework.security.core.userdetails.User(
usernameOrEmail,
user.getPassword(),
authorities
);
}
}
SpringSecurityConfig
Let's create a class SpringSecurityConfig and add the following configuration to it:
@Configuration
@EnableMethodSecurity
@AllArgsConstructor
public class SpringSecurityConfig {
private UserDetailsService userDetailsService;
@Bean
public static PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Bean
SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.csrf(csrf -> csrf.disable())
.authorizeHttpRequests((authorize) -> {
authorize.requestMatchers("/api/auth/**").permitAll();
authorize.anyRequest().authenticated();
}).httpBasic(Customizer.withDefaults());
return http.build();
}
@Bean
public AuthenticationManager authenticationManager(AuthenticationConfiguration configuration) throws Exception {
return configuration.getAuthenticationManager();
}
}
6. Insert SQL Scripts
Before testing Spring security, make sure that you use below SQL scripts below to insert the database into respective tables:
INSERT INTO `users` VALUES
(1,'ramesh@gmail.com','ramesh','$2a$10$5PiyN0MsG0y886d8xWXtwuLXK0Y7zZwcN5xm82b4oDSVr7yF0O6em','ramesh'),
(2,'admin@gmail.com','admin','$2a$10$gqHrslMttQWSsDSVRTK1OehkkBiXsJ/a4z2OURU./dizwOQu5Lovu','admin');
INSERT INTO `roles` VALUES (1,'ROLE_ADMIN'),(2,'ROLE_USER');
INSERT INTO `users_roles` VALUES (2,1),(1,2);
7. Implement Role-Based Authorization
Create a Simple REST APIs and Secure using Admin Role and User Role
@RestController
@RequestMapping("/api/")
public class AdminController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin")
public ResponseEntity<String> helloAdmin(){
return ResponseEntity.ok("Hello Admin");
}
@PreAuthorize("hasRole('USER')")
@GetMapping("/user")
public ResponseEntity<String> helloUser(){
return ResponseEntity.ok("Hello User");
}
}
The above code demonstrates the role-based authorization for RESTful endpoints using Spring Security.
@PreAuthorize("hasRole('ADMIN')"): This is applied to the helloAdmin() method. It specifies that this endpoint can only be accessed by users who have the 'ADMIN'.
@GetMapping("/admin"): This maps HTTP GET requests to /api/admin to the helloAdmin() method. When accessed, it returns a response with "Hello Admin".
@PreAuthorize("hasRole('USER')"): This annotation on the helloUser() method restricts access to users with the 'USER' role. Spring Security enforces this role check before allowing access to the method.
@GetMapping("/user"): Maps HTTP GET requests to /api/user to the helloUser() method. Authorized users with the 'USER' role will receive a "Hello User" response.
Using @PreAuthorize in Spring Boot REST APIs provides a powerful and flexible way to manage access control at the method level. It allows for clear and maintainable security configurations directly in the context of the resources they protect.
8. Testing using Postman
Testing REST API using Admin Role:
Testing REST API using User Role:
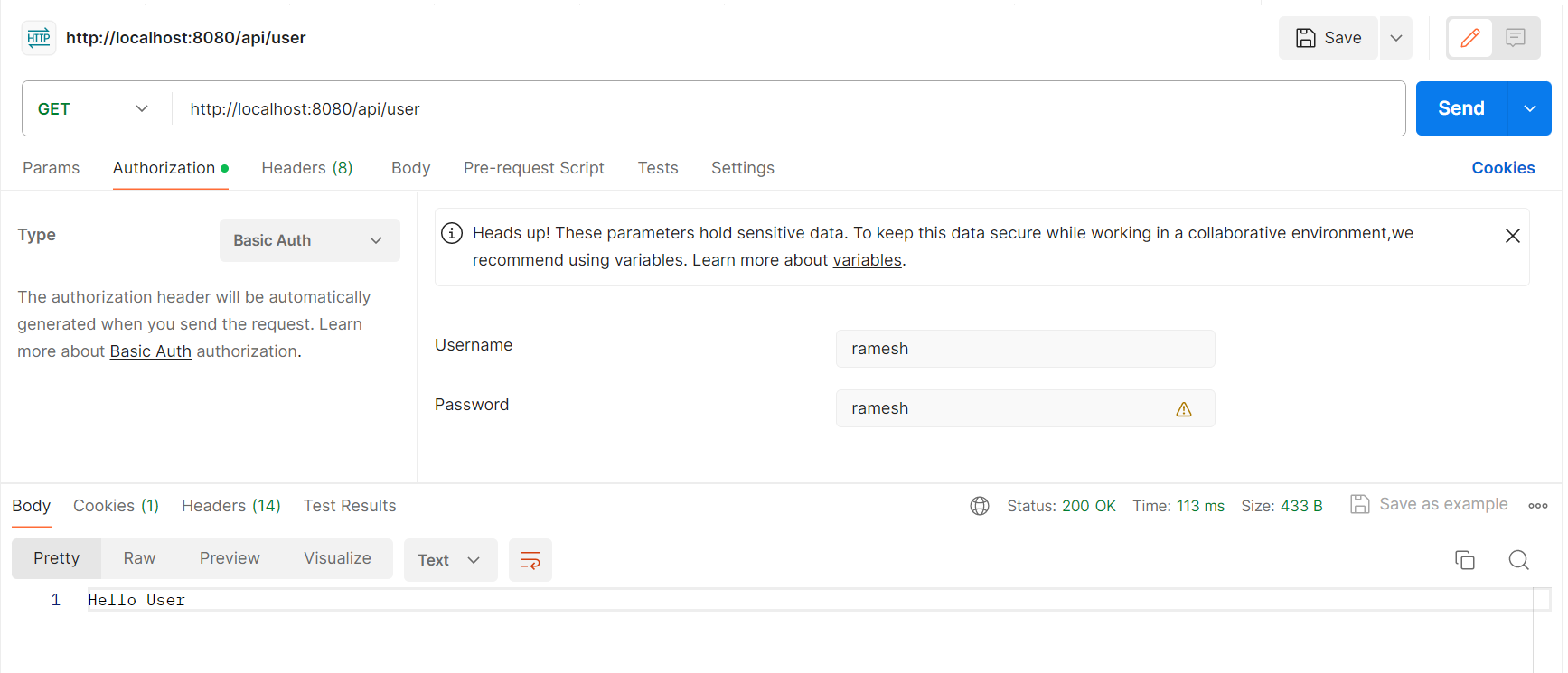
MySQL
Related Spring Boot Source Code Examples
Spring Boot Security Login REST API Example
Spring Boot Security Login and Registration REST API
Role-based Authorization using Spring Boot and Spring Security
Spring Boot JWT Authentication and Authorization Example
Spring Boot Security JWT Example - Login REST API with JWT Authentication
Spring Boot DTO Example
Spring Boot DTO ModelMapper Example
@GetMapping Spring Boot Example
@PostMapping Spring Boot Example
@PutMapping Spring Boot Example
@DeleteMapping Spring Boot Example
@PatchMapping Spring Boot Example
@SpringBootApplication - Spring Boot
Spring Boot Hello World REST API Example
Spring Boot REST API returns Java Bean
Create Spring Boot REST API returns List
Spring Boot REST API with Path Variable
Spring Boot REST API with Request Param
Spring Boot Hibernate MySQL CRUD REST API Tutorial
Spring Boot Real-Time Project Development using Spring MVC + Spring Security + Thymeleaf and MySQL Database
Spring Boot Tutorial - User Login and Registration Backend + Email Verification
Spring Boot JUnit and Mockito Example - Service Layer Testing
Spring Professional Certification Cost
Spring Boot Validate JSON Request Body
Spring Boot One to Many CRUD Example | REST Controller
Spring Boot Project with Controller Layer + Service Layer + Repository/DAO Layer
Spring Boot Reactive MongoDB CRUD Example - WebFlux
Spring Boot Amazon S3 - File Upload Download Delete Example
Spring Boot RabbitMQ Publisher and Consumer Example
Free Spring Boot Open Source Projects for Learning Purposes
Spring Boot + Microsoft SQL Server + Hibernate Example
Spring Boot Hibernate Thymeleaf MySQL CRUD Example
Spring Boot CRUD Example with Spring MVC, Spring Data JPA, ThymeLeaf, Hibernate, MySQL
Spring Boot Hibernate RESTful GET POST PUT and DELETE API Tutorial
Best YouTube Channels to learn Spring Boot
React Spring Boot Example
Spring Boot Groovy Thymeleaf Example Tutorial
Spring Boot Scala Thymeleaf Example Tutorial
Spring Boot Hibernate DAO with MySQL Database Example
Spring Boot PostgreSQL CRUD Example
Spring Boot CRUD Example with MySQL
Spring Boot Starter Parent
Spring Boot JdbcTemplate Example
Spring Boot PayPal Payment Gateway Integration Example
Create Spring Boot REST API
How to Create Spring Boot Application Using Maven
How to Create Spring Boot Application Using Gradle
How to Use Thymeleaf in a Spring Boot Web Application?
How to Enable CORS in a Spring Boot Application?
Spring Boot + Angular 8 CRUD Example
Spring Boot + Angular 9 CRUD Example
Spring Boot + Angular + WebSocket Example
Spring Boot CRUD Application with Thymeleaf
Spring Boot ReactJS CRUD Project - Employee Management App | GitHub
Spring Petclinic ReactJS Project | GitHub
Spring Boot React JWT Authentication Example
Spring Boot React Basic Authentication Example
CRUD Example using Spring Boot + Angular + MySQL
Spring Boot + React + Redux CRUD Example
Spring Boot Project - Sagan
Spring Boot Project - ReactJS Spring Boot CRUD Full Stack Application - GitHub
Spring Boot Project - Spring Initializr
Spring Boot + Angular Project - Employee Management System
Spring Boot Thymeleaf Project - Employee Management System
Spring Boot MVC Project - Blogs Aggregator
Spring Boot Project - Spring Petclinic | GitHub
Spring Boot, Spring Cloud Microservice Project - PiggyMetrics | GitHub
Spring Boot, Spring Security, JWT, React, and Ant Design - Polling App | GitHub
Spring Boot Microservice Project - Shopping Cart App | GitHub
Spring Boot, Spring Cloud Microservice Project - Spring Petclinic App | GitHub
Microservices with Spring Cloud Project | GitHub
Spring Boot Angular Petclinic Project | GitHub
Spring Boot Angular Project - BookStore App | GitHub
React Springboot Microservices Project | GitHub
Spring Boot Microservices, Spring Cloud, and React Project - BookStoreApp | GitHub
Spring Boot + Spring Security + JWT Example
Spring Boot Hibernate Assign UUID Identifiers Example
Spring Boot Angular Project - Reddit Clone Application
Spring Boot Step-by-Step Example
Spring Boot Starters List
Spring Boot E-Commerce Project - Shopizer
Spring Data JPA - save() Method Example
Spring Data JPA - saveAll() Method Example
Spring Data JPA - findById() Method Example
Spring Data JPA - findAll() Method Example
Spring Data JPA - count() Method Example
Spring Data JPA - deleteById() Method Example
Spring Data JPA - delete() Method Example
Spring Data JPA - deleteAll() Method Example
Spring Data JPA - Distinct Query Method Example
Spring Data JPA - GreaterThan Query Method Example
Spring Data JPA - LessThan Query Method Example
Spring Data JPA - Containing Query Method Example
Spring Data JPA - Like Query Method Example
Spring Data JPA - Between Query Method Example
Spring Data JPA - Date Range Between Query Method Example
Spring Data JPA - In Clause Query Method Example
Unit Test Spring Boot GET REST API using JUnit and Mockito
Unit Test Spring Boot POST REST API using JUnit and Mockito
Unit Test Spring Boot PUT REST API using JUnit and Mockito
Unit Test Spring Boot DELETE REST API using JUnit and Mockito
Create REST Client using WebClient for Spring Boot CRUD REST API
Spring Boot WebClient GET Request with Parameters
Spring Boot WebClient POST Request Example
Spring Boot WebClient PUT Request Example
Spring Boot WebClient DELETE Request Example
Spring Boot RestClient GET Request Example
Spring Boot RestClient POST Request Example
Spring Boot RestClient PUT Request Example
Spring Boot RestClient Delete Request Example
Spring Core Annotations with Examples
Spring Boot @Component Example
Spring Boot @Autowired Example
Spring Boot @Qualifier Example
Spring Boot @Primary Example
Spring Boot @Bean Example
Spring Boot @Lazy Example
Spring Boot @Scope Example
Spring Boot @PropertySource Example
Spring Boot @Transactional Example
Spring Boot @Configuration Example
Spring Boot @ComponentScan Example
Spring Boot @Profile Example
Spring Boot @Cacheable Example
Spring Boot @DependsOn Example
Spring Boot @RestController Example
Spring Boot @ResponseBody Example
Spring Boot @GetMapping Example
Spring Boot @PostMapping Example
Spring Boot @PutMapping Example
Spring Boot @DeleteMapping Example
Spring Boot @PatchMapping Example
Spring Boot @PathVariable Example
Spring Boot @ResponseStatus Example
Spring Boot @Service Example
Spring Boot @Repository Example
Spring Boot @RequestParam Example
Spring Boot @SessionAttribute Example
Spring Boot @RequestBody Example
Spring Boot @ExceptionHandler Example
Spring Boot @InitBinder Example
Spring Boot @ModelAttribute Example
Spring Boot @RequestMapping Example
Spring Boot @CrossOrigin Example
Spring Boot @ControllerAdvice Example
Spring Boot @RestControllerAdvice Example
Spring Boot @SpringBootApplication Example
Spring Boot @EnableAutoConfiguration Example
Spring Boot @ConditionalOnClass Example
Spring Boot @SpringBootConfiguration Example
Spring Boot @ConditionalOnProperty Example
Spring Boot @ConditionalOnWebApplication Example
Spring Boot @ConfigurationProperties Example
Spring Boot @Async Example
Spring Boot @Scheduled Example
Spring Boot @SpringBootTest Example
Spring Boot @WebMvcTest Example
Spring Boot @DataJpaTest Example
Spring Boot @EnableDiscoveryClient Example
Spring Boot @EnableFeignClients Example
Spring Boot @RefreshScope Example
Spring Boot @LoadBalanced Example
Spring Boot @Query Example
Spring Boot @Modifying Example
Spring Boot @Param Example
Spring Boot JPA @Transient Example
Spring Boot JPA @Enumerated Example
Spring Boot JPA @Temporal Example
Spring Boot @CreatedBy Example
Spring Boot @LastModifiedDate Example
Spring Boot @IdClass Example
Spring Boot
Spring Security
Comments
Post a Comment