In this tutorial, we show how to add a bootstrap CSS 5 to Thymeleaf templates in Spring boot MVC applications.
We assume that you have already a Spring boot MVC application and you want to add bootstrap CSS 5 to Thymeleaf templates to your existing application.
Create Spring MVC Controller - DemoController
Within a Spring MVC controller, let's create a handler method to handle the request and return the view:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class DemoController {
@GetMapping("bootstrap")
public String demo(){
return "bootstrap";
}
}
Download bootstrap.min.css files
Keep the bootstrap.min.css file in the resources/static folder.
Create Thymeleaf Template
Within the resources/templates folder, let's create a Thymeleaf template named "bootstrap" and add the below content to it:
<!DOCTYPE html>
<html lang="en"
xmlns:th="http://www.thymeleaf.org"
>
<head>
<meta charset="UTF-8">
<title>Hello</title>
<link th:href="@{/css/bootstrap.min.css}" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="text-center">Page Heading</h1>
<table class="table table-striped table-bordered">
<thead class="table-dark">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
</thead>
<tbody>
<tr>
<td>Ramesh</td>
<td>Fadatare</td>
</tr>
<tr>
<td>Umesh</td>
<td>Fadatare</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Note that we have included a bootstrap CSS file:
<link th:href="@{/css/bootstrap.min.css}" rel="stylesheet">
Using Bootstrap 5 CDN Link
You can also use the bootstrap 5 remote CDN link to use the bootstrap in a Thymeleaf HTML file:
<!DOCTYPE html>
<html lang="en"
xmlns:th="http://www.thymeleaf.org"
>
<head>
<meta charset="UTF-8">
<title>Hello</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<div class="container">
<h1 class="text-center">Page Heading</h1>
<table class="table table-striped table-bordered">
<thead class="table-dark">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
</thead>
<tbody>
<tr>
<td>Ramesh</td>
<td>Fadatare</td>
</tr>
<tr>
<td>Umesh</td>
<td>Fadatare</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Bootstrap 5 CDN link:
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
Run Spring Boot Application
Hit this URL in the browser:
http://localhost:8080/bootstrap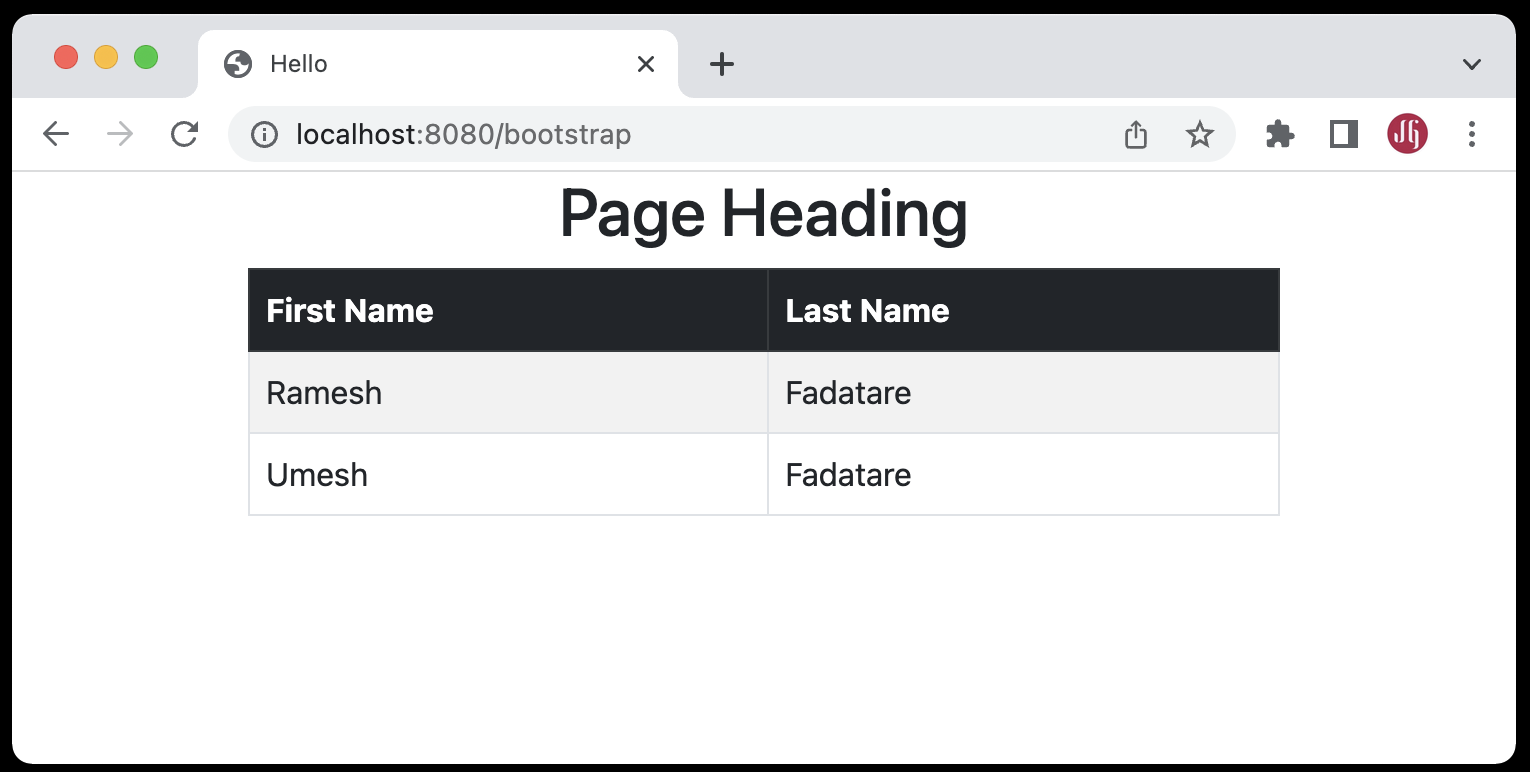
Related Java Source Code Examples:
Java Record Class Example
Java Text Blocks Example
Java Record Builder Pattern Example
Java Record Constructor Example
Java Record Constructor Validation Example
Java Record Serializable Example
Java Record Getter Example
Java Record Default Constructor
Java Records CRUD (Create, Read, Update, Delete) Example
Java Record Custom Methods
Java Record Custom toString
Java Record Custom equals and hashCode
Java Records with Spring Boot CRUD Example
Java Record Implement Interface Example
Java Switch Expressions Example
Java Pattern Matching for instanceof
Java Sealed Class Example
Java Sealed Class vs Final Class
Java Sealed Class Record Example
Java Sealed Class Generic Example
Java Sealed Class Enum Example
Java Sealed Class Switch Example
Java var Keyword Example
Java CompactNumberFormat Class Example
Java Vector API Example
Pattern Matching for Switch Example
Collectors.teeing() in Stream API Example
Java FileSystems.newFileSystem() Method Example
Java Files.readString() and Files.writeString() Example
Java String isBlank Example
Java String strip Example
Java String repeat Example
Java String lines Example
Java String stripIndent Example
Java Binary Search (Recursive and Iterative) Program
Java Happy Birthday Program
Java Program That Calculates the Age of a Person
Java Logical And Or Xor Examples
Java Format Date and Time Examples
Java Convert String to Date and Time
Java Convert Roman to Integer Number
Java Convert Octal to HexaDecimal
Java Convert Octal to Decimal
Java Convert Integer Number to Roman Number
Java Convert Hexadecimal to Binary
Java Convert Decimal to Octal
Java Convert Decimal to Binary
Java Convert Binary to Decimal
Java Convert Binary to an Octal
Java Convert Binary to a Hexadecimal
Java Convert a Unix Timestamp to Date Time
Java Check If Two Integer Arrays are Equals
Java program that removes all white spaces from the given string
Java 8 program that counts the number of vowels and consonants in a given string
Java 8 program that reverses words of a given string
Java Program to Count Number of Digits in an Integer
Java Program Fibonacci Series Using Recursion
Java Program to Find Factorial of a Number
Java program to calculate the area of Triangle
Java Program to Calculate Area of Square
Java Program to Calculate Area of Rectangle
Java Program to find the Smallest of three numbers using Ternary Operator
Java Program to Find Largest of Three Numbers
Java Program to Find GCD of Two Numbers
Java Program to Check Armstrong Number
Java Program to Generate Random Number
Java Program to Check if Number is Positive or Negative
Java program to check prime number
Java Program to Calculate Simple Interest
Java Program to Swap Two Numbers Without using a Temporary Variable
Java Program to Swap Two Numbers
Java Program to Find ASCII Value of a Character
Java Program to Check Whether an Alphabet is Vowel or Consonant
Java Program to Check Leap Year
Java Program to Check Even or Odd Number
Java Program to Add Two Numbers
Java Program to Swap Two Strings Without Using Third Variable
Java Program to Swap Two Strings with Third Variable
How to Get All Digits from String in Java
Find Duplicate Number in Array in Java
How to Get Current Working Directory in Java?
Check Palindrome String in Java
Java Program to Create Pyramid Of Numbers
Create a String object in Java Example
Java String valueOf() Method Example
Java String.trim() Method Example
Java String.toUpperCase() Method Example
Java String toLowerCase() Method Example
Java String toCharArray() Method Example
Java String substring() Method Example
Java String.startsWith() Method Example
Java String split() Method Example
Java String.replaceFirst() Method Example
Java String.replaceAll() Method Example
Java String replace() Method Example
Java String regionMatches() Method Example
Java String length() Method Example
Java String lastIndexOf() Method Example
Java String intern() Method Example
Java String indexOf() Method Example
Java String getChars() Method Example
Java String getBytes() Method Example
Java String equals() and equalsIgnoreCase() Example
Java String.endsWith() Method Example
Java String.contentEquals() Method Example
Java String.contains() Method Example
Java String concat() Method Example
What's the Simplest Way to Print a Java Array?
Merge two String Arrays Example
Checks If a String is Not Empty and Not Null in Java
Reverse a String in Java Using StringBuilder
Capitalize the First Letter of a String in Java Example
Java String codePointAt() Method Example
Java String Compare Using equals() Method
Sort String in Descending Order in Java
Sort String in Ascending Order in Java
How to Get All Digits from String in Java
Java String getDigits() Utility Method
Java String isAlphanumeric() Utility Method
Java String isAlpha() Utility Method
Java String countMatches() Utility Method
Java String mergeStringArrays(String array1[], String array2[]) Utility Method Example
Java String quote( String str) Utility Method
Java arrayToMap(String[][] array) Utility Method
Java String fromBufferedReader() Utility Method - Converts a BufferedReader into a String
Java String isNumericSpace() Utility Method
Java String isNumeric() Utility Method
Java String isAlphanumericSpace() Utility Method
Java String isAlphaSpace(() Utility Method
Java trimArrayElements() Utility Method
Java trimArrayElements() Utility Method
Java String containsWhitespace() Utility Method
Java String isBlank() Utility Method
Java String isAnyEmpty() Utility Method
Java String isNotEmpty() Utility Method
Java String isEmpty() Utility Method
Java String Uppercase First Character Example
Java String uppercaseFirstChar() Utility Method
Java String trimTrailingCharacter() Utility Method
Java String trimLeadingCharacter() Utility Method
Java String trimTrailingWhitespace() Utility Method
Java String trimLeadingWhitespace() Utility Method
Java String trimAllWhitespace() Utility Method
Java String trimWhitespace() Utility Method
Java StringBuilder trimToSize() Method Example
Java StringBuilder substring() Method Example
Java StringBuilder setLength() Method Example
Java StringBuilder reverse() Method Example
Java StringBuilder lastIndexOf() Method Example
Java StringBuilder insert() Method Example
Java StringBuilder indexOf() Method Example
Java StringBuilder getChars() Method Example
Java StringBuilder ensureCapacity() Method Example
Java StringBuilder deleteCharAt() Method Example
Java StringBuilder delete() Method Example
Java StringBuilder codePointBefore() Method Example
Java StringBuilder codePointAt Method Example
Java StringBuilder capacity() Method Example
Java StringBuilder append() Method Example
Java Convert Integer to String Example
Java Convert Float to String Example
Java Convert Double to String Example
Java Convert Short to String Example
Java Convert Long to String Example
Java Convert Character to String Example
Java Convert Byte to String Example
Java Convert Boolean to String Example
Java Convert String To Short Example
Java Convert String To Long Example
Java Convert String To Integer Example
Java Convert String To Float Example
Java Convert String To Double Example
Java Convert String To Byte Example
Java Convert String To Boolean Example
Clear ArrayList in Java Example
Java ArrayList add(), get() and set() Method Example
Iterate over ArrayList Using forEach Java
Iterate over ArrayList using Iterator in Java
Java ArrayList indexOf() and lastIndexOf() Example
Search an Element in an ArrayList in Java
Java ArrayList removeAll() Method Example
Java ArrayList remove() Method Example
How to Iterate over ArrayList in Java
How to Remove Element from ArrayList in Java
How to Access Elements of ArrayList in Java
Create ArrayList from Another ArrayList in Java
How to Create an ArrayList and Add Elements to It
Java ArrayList subList() Example
Get Index of Elements in ArrayList Example
Java ArrayList removeIf() Example
Copying part of Array in Java Example
Sorting an Array using Arrays.sort() Method
Check Two Arrays are Deeply Equal
Java Arrays.copyOf() Example - Copying an Array
Java Arrays.binarySearch() Example - Searching an Array
Java Arrays.asList() Example - Convert Array to ArrayList
Sort String in Ascending Order in Java
Sort String in Descending Order in Java
Sort Integer in Ascending Order in Java Example
Sort Integer in Descending Order in Java Example
Sort User-Defined Objects in Ascending Order in Java
Sort User-Defined Objects in Descending Order in Java
Sort User-Defined Objects using Comparator Interface
Java LinkedList add(), addFirst(), addLast() and addAll() Example
Iterate LinkedList using forEach() Method Example
Iterate through a LinkedList using an Iterator in Java
Iterate or Loop Through Elements LinkedList in Java Example
Search Elements of LinkedList in Java with Example
Remove First and Last Elements of LinkedList in Java
Get Elements from LinkedList Java Example
Java LinkedList remove(), removeFirst(), removeLast(), removeIf() and clear() Example
Create HashSet in Java
Iterating over a HashSet using Iterator
Iterate over HashSet in Java
Remove Element from HashSet in Java
Create HashSet in Java
Iterating over a LinkedHashSet using forEach() Method Example
Iterate over LinkedHashSet using Iterator in Java Example
Iterate over LinkedHashSet in Java Example
Remove all Elements from LinkedHashSet in Java Example
Remove Element from LinkedHashSet Example
Check If an Element Exists in Java LinkedHashSet Example
Create LinkedHashSet in Java Example
Create TreeSet in Java Example
Iterating over a TreeSet using Java 8 forEach() Method
Iterate over a TreeSet using Iterator in Java Example
Iterate over TreeSet in Java Example
Remove All Elements from Java TreeSet Example
Remove First and Last Element from TreeSet in Java
Remove Element from TreeSet in Java Example
Check If an Element Exists in Java TreeSet Example
Java TreeSet add(), first() and last() Method Example
Java Convert ArrayList to Stack Example
Java Convert Array to Stack Example
Java Stack push() Method Example
Java Stack empty() Method Example
Java Stack peek() Method Example
Java Stack search() Method Example
Java Stack pop() Method Example
Java Deque and ArrayDeque Examples
Iterating over a Queue in Java Example
Java Queue isEmpty(), size(), element() and peek() Example
Java Queue Interface Example
Java Priority Queue of User Defined Objects Example
Java Create a Priority Queue with a Custom Comparator
Create a Priority Queue in Java Example
HashMap Stream and Filtering Example
Java HashMap Iteration over Values Example
Java HashMap Iteration over Keys
Java HashMap replace() Example
Java HashMap size Example
Iterate over a HashMap Java using Lambda
Iterate over a HashMap Java 8 forEach
Iterating over a HashMap using Iterator in Java
Iterate over HashMap in Java
How to Store Employee Objects in HashMap
Remove Entry from HashMap Java
Check If Value Exists in HashMap Java Example
Check If Key Exists in HashMap Java Example
Create a HashMap in Java Example
How to Delete Data from MySQL Table using Java
How to Insert Data in MySQL Table using Java
How to Create Database Table in Java
JDBC DriverManager Example
JDBC PreparedStatement Example
JDBC Statement Example
Java MySQL DataSource Example
Update Query in MySQL using Java PreparedStatement
Login Example with JSP + Servlet + JDBC + MySQL Database
JSP + JDBC + MySQL Example Tutorial
JSP Form Example with JDBC and MySQL Database
Java JDBC CRUD Example - SQL Insert, Select, Update, and Delete Examples
Java Swing JDBC MySQL Database Example
Java JDBC Connection to HSQLDB Database
JDBC Connection to H2 Database Example
JDBC Connection to PostgreSQL Example
JDBC Connection to Oracle Database Example
JDBC Transaction Management Example
JDBC Delete Query Example
JDBC Select Query Example
JDBC Update Query Example
JDBC Insert Multiple Rows Example
JDBC Create a Table Example
What are different types of JDBC Drivers
Advantages of Hibernate Framework over JDBC
Java PostgreSQL Example
Java H2 Insert Record Example
Java H2 Embedded Database Example
Java H2 Create Table Example
Java Simple Lambda Expression Example
Java Lambda Expressions Multiple Statements
Java Lambda Expressions forEach Example
Java Lambda Expression with Multiple Parameters Example
Java Lambda Expression Runnable Example
Java Lambda Expression Comparator Example
Refactoring Factory Design Pattern with Lambdas
Refactoring Chain of Responsibility Pattern with Lambdas
Refactoring Observer Design Pattern with Lambdas
Refactoring Strategy Design Pattern with Lambdas
Iterate HashMap with forEach() + Lambda Expression Example
Iterate HashSet with forEach() + Lambda Expression Example
Iterate ArrayList with forEach() + Lambda Expression Example
Java method reference to a constructor example
Java method reference to static method example
Java method reference to an instance method of an object example
Java method reference to an instance method of an arbitrary object of a particular type
Java 8 - Create Stream Object from Arrays Example
Java 8 Creating a Stream from an Array
Java 8 - Create Stream Object from Collection, List, Set
Java 8 Stream - Sort List of String objects in Descending Order Example
Java 8 Stream - Sort List of String objects in Ascending Order Example
How to convert a Java 8 Stream to an Array?
Java 8 Stream - allMatch(),anyMatch() and noneMatch() Example
Java 8 Stream - Sort List of Objects by Multiple Fields
Java 8 Stream - Sort List of Custom Objects in Ascending and Descending Order
Java 8 - Stream filter(), forEach(), collect() and Collectors.toList() Example
Java Stream filter null values example
Java Stream filter map by values example
Java Stream filter map by keys example
Java 8 Collectors.counting() Example
Java 8 Collectors.averagingDouble() Example
Java 8 Collectors.toSet() Example
Java 8 Collectors.toList() Example
Java 8 Collectors.joining() example
Java 8 Stream multiple filter operations
Java 8 Stream filter map by keys
Java 8 Stream filter map by values
Java 8 Stream collect to list example
Java 8 Stream Collectors.counting() exmaple
Java 8 Collectors.collectingAndThen() example
Java 8 Collectors.toList() Example
Java 8 Collectors.minBy() and Collectors.maxBy() example
Java Stream filter() Example
Java Stream map() Example
Java Stream flatMap() Example
Java Stream distinct() Example
Java Stream limit() Example
Java Stream peek() Example
Java Stream anyMatch() Example
Java Stream allMatch() Example
Java Stream noneMatch() Example
Java Stream collect() Example
Java Stream count() Example
Java Stream findAny() Example
Java Stream findFirst() Example
Java Stream forEach() Example
Java Stream min() Example
Java Stream max() Example
Java Stream reduce() Example
Java Stream toArray() Example
Java Function Functional Interface Example
Java Supplier Functional Interface Example
Java Consumer Example
Java BiConsumer Example
Java BiFunction Example
Java Predicate Example
Java 8 LongConsumer Example
Java 8 IntConsumer Example
Java 8 DoubleConsumer Example
Java StringJoiner Example
Create Optional Class Object in Java - empty(), of(), ofNullable() Methods
Optional get() Method - Get Value from Optional Object in Java
Optional isPresent() Method Example
Optional orElse() Method Example
Optional orElseGet() Method Example
Optional orElseThrow() Method Example
Optional filter() and map() Method Examples
Java 8 program that counts the number of vowels and consonants in a given string
Java 8 program that reverses words of a given string
Java 8 - Copy List into Another List Example
Check Whether the Given Year is Leap Year or Not in Java
Java 8 forEach() Array Example
Java 8 forEach() Map Example
Java 8 forEach() Set Example
Java 8 forEach() List Example
Getting current date and time with Clock
Convert LocalDate to Date in Java
Convert LocalDateTime into Date in Java
Connvert java.util.Date to java.time.LocalDateTime Example
Convert java.util.Date to java.time.LocalDate Example
Duration Between Two LocalDate in Java
Java Convert ZonedDateTime to String Example
Convert String to ZonedDateTime in Java
Compare ZonedDateTime Objects in Java
How to Get Hour, Minute, Second from ZonedDateTime in Java
Convert ZonedDateTime to LocalTime Example
Convert ZonedDateTime to LocalDateTime in Java
Java ZonedDateTime now() and of() Method Example
Convert LocalDateTime to LocalTime in Java
Convert LocalDateTime to LocalDate in Java
Compare LocalDateTime Objects in Java
Java LocalDateTime - Get Hour, Minute and Second
How to Get Day Month and Year from LocalDateTime in Java
Java LocalDateTime now() Method Example
How to Get Current Date Time in Java
Convert LocalDate to String in Java
Convert String to LocalDate in Java
Java LocalDate isLeapYear() Method Example
How to Get Number of Days from Month and Year using LocalDate in Java
Compare Java LocalDate Objects Example
How to Add Days Weeks Months and Years to LocalDate in Java
How to get Day Month and Year from LocalDate in Java
Java LocalDate now() Method Example - Get Current Date and Specific Date
Convert LocalTime to String in Java
Convert String to LocalTime in Java
Java LocalTime isBefore() and isAfter() Method Example
How to Compare Time in Java
Java 8 - LocalTime getHour(), getMinute(), getSecond() and getNano() Example
Java LocalTime now() Method Example - Get Current Time and Specific Time
Java 8 Get Local Date Time in All Available Time Zones
How to get year, month, day, hours, minutes, seconds and milliseconds of LocalDateTime in Java 8?
How to get year, month, day, hours, minutes, seconds and milliseconds of Date in Java?
Difference between Two Dates in Java
Create LocalDateTime from LocalDate and LocalTime in Java
Java
Related Spring Boot Source Code Examples
Spring Boot Security Login REST API Example
Spring Boot Security Login and Registration REST API
Role-based Authorization using Spring Boot and Spring Security
Spring Boot JWT Authentication and Authorization Example
Spring Boot Security JWT Example - Login REST API with JWT Authentication
Spring Boot DTO Example
Spring Boot DTO ModelMapper Example
@GetMapping Spring Boot Example
@PostMapping Spring Boot Example
@PutMapping Spring Boot Example
@DeleteMapping Spring Boot Example
@PatchMapping Spring Boot Example
@SpringBootApplication - Spring Boot
Spring Boot Hello World REST API Example
Spring Boot REST API returns Java Bean
Create Spring Boot REST API returns List
Spring Boot REST API with Path Variable
Spring Boot REST API with Request Param
Spring Boot Hibernate MySQL CRUD REST API Tutorial
Spring Boot Real-Time Project Development using Spring MVC + Spring Security + Thymeleaf and MySQL Database
Spring Boot Tutorial - User Login and Registration Backend + Email Verification
Spring Boot JUnit and Mockito Example - Service Layer Testing
Spring Professional Certification Cost
Spring Boot Validate JSON Request Body
Spring Boot One to Many CRUD Example | REST Controller
Spring Boot Project with Controller Layer + Service Layer + Repository/DAO Layer
Spring Boot Reactive MongoDB CRUD Example - WebFlux
Spring Boot Amazon S3 - File Upload Download Delete Example
Spring Boot RabbitMQ Publisher and Consumer Example
Free Spring Boot Open Source Projects for Learning Purposes
Spring Boot + Microsoft SQL Server + Hibernate Example
Spring Boot Hibernate Thymeleaf MySQL CRUD Example
Spring Boot CRUD Example with Spring MVC, Spring Data JPA, ThymeLeaf, Hibernate, MySQL
Spring Boot Hibernate RESTful GET POST PUT and DELETE API Tutorial
Best YouTube Channels to learn Spring Boot
React Spring Boot Example
Spring Boot Groovy Thymeleaf Example Tutorial
Spring Boot Scala Thymeleaf Example Tutorial
Spring Boot Hibernate DAO with MySQL Database Example
Spring Boot PostgreSQL CRUD Example
Spring Boot CRUD Example with MySQL
Spring Boot Starter Parent
Spring Boot JdbcTemplate Example
Spring Boot PayPal Payment Gateway Integration Example
Create Spring Boot REST API
How to Create Spring Boot Application Using Maven
How to Create Spring Boot Application Using Gradle
How to Use Thymeleaf in a Spring Boot Web Application?
How to Enable CORS in a Spring Boot Application?
Spring Boot + Angular 8 CRUD Example
Spring Boot + Angular 9 CRUD Example
Spring Boot + Angular + WebSocket Example
Spring Boot CRUD Application with Thymeleaf
Spring Boot ReactJS CRUD Project - Employee Management App | GitHub
Spring Petclinic ReactJS Project | GitHub
Spring Boot React JWT Authentication Example
Spring Boot React Basic Authentication Example
CRUD Example using Spring Boot + Angular + MySQL
Spring Boot + React + Redux CRUD Example
Spring Boot Project - Sagan
Spring Boot Project - ReactJS Spring Boot CRUD Full Stack Application - GitHub
Spring Boot Project - Spring Initializr
Spring Boot + Angular Project - Employee Management System
Spring Boot Thymeleaf Project - Employee Management System
Spring Boot MVC Project - Blogs Aggregator
Spring Boot Project - Spring Petclinic | GitHub
Spring Boot, Spring Cloud Microservice Project - PiggyMetrics | GitHub
Spring Boot, Spring Security, JWT, React, and Ant Design - Polling App | GitHub
Spring Boot Microservice Project - Shopping Cart App | GitHub
Spring Boot, Spring Cloud Microservice Project - Spring Petclinic App | GitHub
Microservices with Spring Cloud Project | GitHub
Spring Boot Angular Petclinic Project | GitHub
Spring Boot Angular Project - BookStore App | GitHub
React Springboot Microservices Project | GitHub
Spring Boot Microservices, Spring Cloud, and React Project - BookStoreApp | GitHub
Spring Boot + Spring Security + JWT Example
Spring Boot Hibernate Assign UUID Identifiers Example
Spring Boot Angular Project - Reddit Clone Application
Spring Boot Step-by-Step Example
Spring Boot Starters List
Spring Boot E-Commerce Project - Shopizer
Spring Data JPA - save() Method Example
Spring Data JPA - saveAll() Method Example
Spring Data JPA - findById() Method Example
Spring Data JPA - findAll() Method Example
Spring Data JPA - count() Method Example
Spring Data JPA - deleteById() Method Example
Spring Data JPA - delete() Method Example
Spring Data JPA - deleteAll() Method Example
Spring Data JPA - Distinct Query Method Example
Spring Data JPA - GreaterThan Query Method Example
Spring Data JPA - LessThan Query Method Example
Spring Data JPA - Containing Query Method Example
Spring Data JPA - Like Query Method Example
Spring Data JPA - Between Query Method Example
Spring Data JPA - Date Range Between Query Method Example
Spring Data JPA - In Clause Query Method Example
Unit Test Spring Boot GET REST API using JUnit and Mockito
Unit Test Spring Boot POST REST API using JUnit and Mockito
Unit Test Spring Boot PUT REST API using JUnit and Mockito
Unit Test Spring Boot DELETE REST API using JUnit and Mockito
Create REST Client using WebClient for Spring Boot CRUD REST API
Spring Boot WebClient GET Request with Parameters
Spring Boot WebClient POST Request Example
Spring Boot WebClient PUT Request Example
Spring Boot WebClient DELETE Request Example
Spring Boot RestClient GET Request Example
Spring Boot RestClient POST Request Example
Spring Boot RestClient PUT Request Example
Spring Boot RestClient Delete Request Example
Spring Core Annotations with Examples
Spring Boot @Component Example
Spring Boot @Autowired Example
Spring Boot @Qualifier Example
Spring Boot @Primary Example
Spring Boot @Bean Example
Spring Boot @Lazy Example
Spring Boot @Scope Example
Spring Boot @PropertySource Example
Spring Boot @Transactional Example
Spring Boot @Configuration Example
Spring Boot @ComponentScan Example
Spring Boot @Profile Example
Spring Boot @Cacheable Example
Spring Boot @DependsOn Example
Spring Boot @RestController Example
Spring Boot @ResponseBody Example
Spring Boot @GetMapping Example
Spring Boot @PostMapping Example
Spring Boot @PutMapping Example
Spring Boot @DeleteMapping Example
Spring Boot @PatchMapping Example
Spring Boot @PathVariable Example
Spring Boot @ResponseStatus Example
Spring Boot @Service Example
Spring Boot @Repository Example
Spring Boot @RequestParam Example
Spring Boot @SessionAttribute Example
Spring Boot @RequestBody Example
Spring Boot @ExceptionHandler Example
Spring Boot @InitBinder Example
Spring Boot @ModelAttribute Example
Spring Boot @RequestMapping Example
Spring Boot @CrossOrigin Example
Spring Boot @ControllerAdvice Example
Spring Boot @RestControllerAdvice Example
Spring Boot @SpringBootApplication Example
Spring Boot @EnableAutoConfiguration Example
Spring Boot @ConditionalOnClass Example
Spring Boot @SpringBootConfiguration Example
Spring Boot @ConditionalOnProperty Example
Spring Boot @ConditionalOnWebApplication Example
Spring Boot @ConfigurationProperties Example
Spring Boot @Async Example
Spring Boot @Scheduled Example
Spring Boot @SpringBootTest Example
Spring Boot @WebMvcTest Example
Spring Boot @DataJpaTest Example
Spring Boot @EnableDiscoveryClient Example
Spring Boot @EnableFeignClients Example
Spring Boot @RefreshScope Example
Spring Boot @LoadBalanced Example
Spring Boot @Query Example
Spring Boot @Modifying Example
Spring Boot @Param Example
Spring Boot JPA @Transient Example
Spring Boot JPA @Enumerated Example
Spring Boot JPA @Temporal Example
Spring Boot @CreatedBy Example
Spring Boot @LastModifiedDate Example
Spring Boot @IdClass Example
Spring Boot
Thymeleaf
Comments
Post a Comment